Google Tag Manager comes with a lot of useful build-in variables to use in triggers and tags, like the click-variables: “Click Element”, “Click ID” and “Click Classes”. These variables are useful when you want to base a trigger or tag on the exact element that is being clicked. However, what if you want to trigger a tag on a click based on one of the parent element’s characteristics of the clicked element?
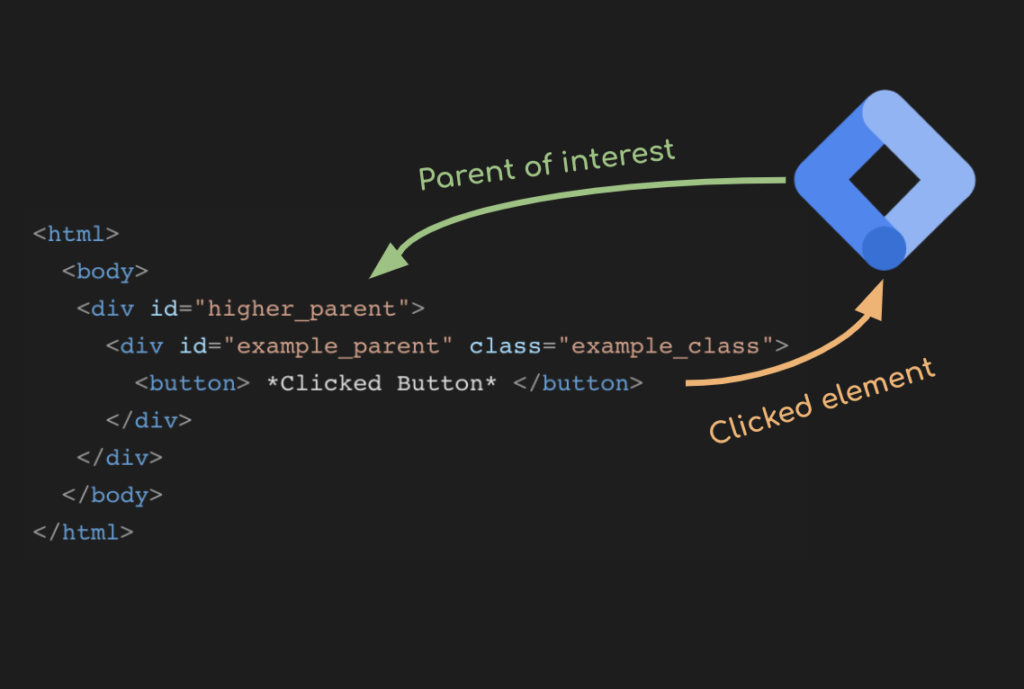
We’re going to create a GTM Utility/function variable which gives you more abilities to configure triggers and tags based on attributes from HTML elements higher in the DOM structure from the clicked element.
Parent HTML Elements
With Parent HTML elements, I mean the HTML elements which are higher in the HTM in the DOM (Document Object Model), or less “nested” in the DOM compared to the clicked element.
For example, in the code below I clicked the button
element. The div
element with the id “example_parent” in which this button element is inside of, is the button’s direct parent element. The div element one level higher in the DOM, is the button’s indirect parent
<html>
<body>
<div id="higher_parent">
<div id="example_parent" class="example_class">
<button> *Clicked Button* </button>
</div>
</div>
</body>
</html>
Use cases
Knowing whether a clicked element is inside of a specific parent can be really userful for differentiating user behavior with GTM. Use cases include:
- Checking if a clicked element is within a pop-up, promotional wrapper or warning message to differentiate the tracking of that click event
- Excluding the tracking of clicks outside of specific parents in triggers
- Including only the tracking of clicks within specific parent elements in triggers
- …
Let’s assume you track all the link clicks on your website. Now you want to differentiate these clicks based on the dropdown menu in which the link click was triggered.
E.g. We want to track the link clicks on Amazon.com’s account dropdown with a different event name. For this to work we would only need to have a variable that will return to us wether the link click happened in the dropdown or not.
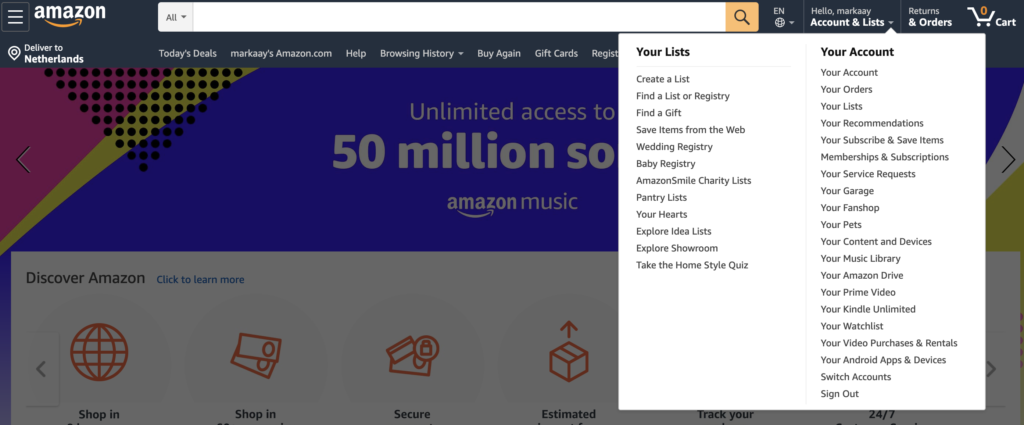
So that is what we’re going to create; A utility function that checks if the clicked element is within a specific parent element and returns a boolean value.
The GTM utility variable
The utility variable we are going to create, will check parent elements based on their classes. So, this function will return true or false if the clicked element is within a parent element which contains a specific class.
Create a new custom javascript variable and paste in the code below. Save the GTM variable (e.g. “function.withinParentWithClass”) and you’re ready to use this function 🙂
function(){
//return an utility function
return function(click_element, parent_class_string)
//use a loop function to check all parents up the DOM tree
function isWithinParentWithClass(element, class_name) {
if (element.className.split(' ').indexOf(class_name)>=0) return true;
return element.parentNode && isWithinParentWithClass(element.parentNode, class_name);
}
//Return true if click_element is within parent with specified class
return isWithinParentWithClass(click_element, parent_class_string);
}
}
How to use:
The function above can be called in other GTM variables, and tags to get true or false values. The function can be called as followed:
{{function.withinParentWithClass}}({{Click Element}}, 'example_class');
The first input value of the function must be the build-in {{Click Element}}
variable. The second input value should be the class for which the utility function should look for in parent elements.
To really turn the utility function into variables which you can use in GTM triggers, you’ll need to wrap the utility function into another GTM custom javascript variable as followed:
function(){
return {{function.withinParentWithClass}}({{Click Element}}, 'example_class');
}
This new custom javascript variable will return true if the {{Click Element}} is within one of the parent elements with the provided class, “example_class”.
If we take a look back at the HTML example at the top of this reading, the new custom javascript variable will return true if we click the button element.
Controlling for other parent attributes
In the example above, I’m using the parent classes to determine whether a click happened within those parent elements. However, you can also do this with other click element attributes. Attributes like the id, value, data-attributes and even custom HTML attributes, can be used similarly to the use of classes.
If there’s any interest in these other methods, leave a comment.